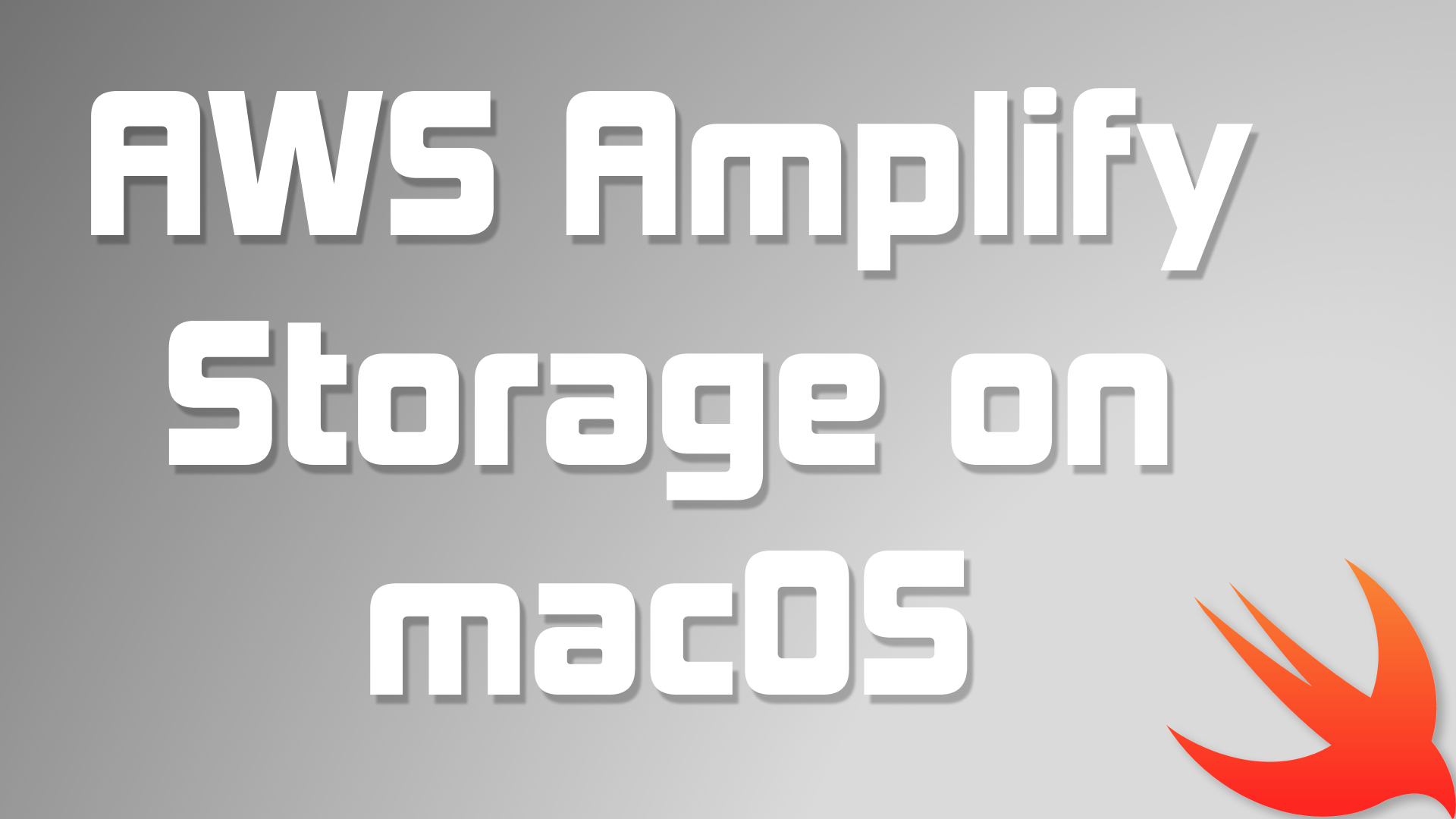
Getting Started with AWS Amplify Storage on macOS: A Step-by-Step Guide for iOS and macOS Developers
If you're an iOS or macOS developer looking to add cloud storage capabilities to your app, AWS Amplify Storage is an excellent option to consider. AWS Amplify Storage is a service provided by Amazon Web Services that allows developers to easily store, retrieve, and manage data in the cloud. In this blog post, we'll guide you through the steps necessary to get started with AWS Amplify Storage on macOS.
Step 1: Create a New Amplify Project To get started with AWS Amplify Storage, you'll need to create a new Amplify project. Open your terminal and navigate to the directory where you want to create your project. Then, run the following command:
$ amplify init
This will prompt you to answer a few questions about your project, such as the project name and the type of app you're building. Once you've answered all the questions, Amplify will create a new project directory with all the necessary files and configurations.
Step 2: Add Storage to Your Amplify Project Now that you've created a new Amplify project, you can add storage capabilities to it. Run the following command to add AWS Amplify Storage to your project:
$ amplify add storage
This will prompt you to choose the type of storage you want to add. Choose the option that best fits your needs and follow the prompts to configure your storage.
Step 3: Use AWS Amplify Storage in Your App Once you've added storage to your Amplify project, you can start using it in your app. Amplify provides a simple and intuitive API that you can use to upload, download, and manage your data in the cloud.
Here's an example of how you can use the Amplify Storage API to upload a file to your storage:
import Amplify
import AWSS3StoragePlugin
// Initialize Amplify
Amplify.configure()
// Upload a file to S3
let dataString = "My Data"
let data = Data(dataString.utf8)
let uploadTask = Amplify.Storage.uploadData(
key: "ExampleKey",
data: data
)
Task {
for await progress in await uploadTask.progress {
print("Progress: \(progress)")
}
}
let value = try await uploadTask.value
print("Completed: \(value)")
In this example, we're importing the Amplify and AWSS3StoragePlugin frameworks, initializing Amplify, and using the Storage.uploadFile() method to upload a file to Amazon S3. Once the upload is complete, we're printing a success message or an error message depending on the result.
You can also use the Amplify Storage API to download files, list files, and delete files from your storage. Here's an example of how to list all the files in your storage:
let listResult = try await Amplify.Storage.list()
listResult.items.forEach { item in
print("Key: \(item.key)")
}
This code imports the Amplify framework, initializes Amplify, and uses the Storage.list() method to list all the files in your storage. Once the list is returned, the code prints the name of each file.
Conclusion
In this blog post, we've covered the steps necessary to get started with AWS Amplify Storage on macOS. We've walked through the process of creating a new Amplify project, adding storage capabilities to it, and using the Amplify Storage API to upload, download, and manage files in the cloud. By following these steps, you can add powerful cloud storage capabilities to your iOS and macOS apps in no time.
Remember to always follow best practices for security and access control when using AWS Amplify Storage in your app. With the right approach, AWS Amplify Storage can help you build secure, scalable, and highly available apps that leverage the power of the cloud.